Local web = require('web') function printtable(t) for k,v in pairs(t) do print(k,v) end end results = web.query('Lua site:en.wikipedia.org') printtable(results). This is a general reference for scripting in Lua on Roblox. This cheat sheet is intended for beginner scripters. Access this cheat sheet here. Roblox Gamepad Input. This is a quick reference for implementing gamepad (controller) input on Roblox. This cheat sheet is. Local web = require('web') function printtable(t) for k,v in pairs(t) do print(k,v) end end results = web.query('Lua site:en.wikipedia.org') printtable(results).

This is a function 'cheat sheet' for Apollo extension by YellowAfterlife.
The extension can be acquired from GM:Marketplace or itch.io.
For questions/support, use forums (itch.io, GM forums), or send me an email.
A most up-to-date version of the manual is always available online.
The extension is currently available for Windows, Mac (GMS2 only), and Linux.
Click on sections to expand/collapse them.
Quick display controls: Categories · Sections · Everything ·


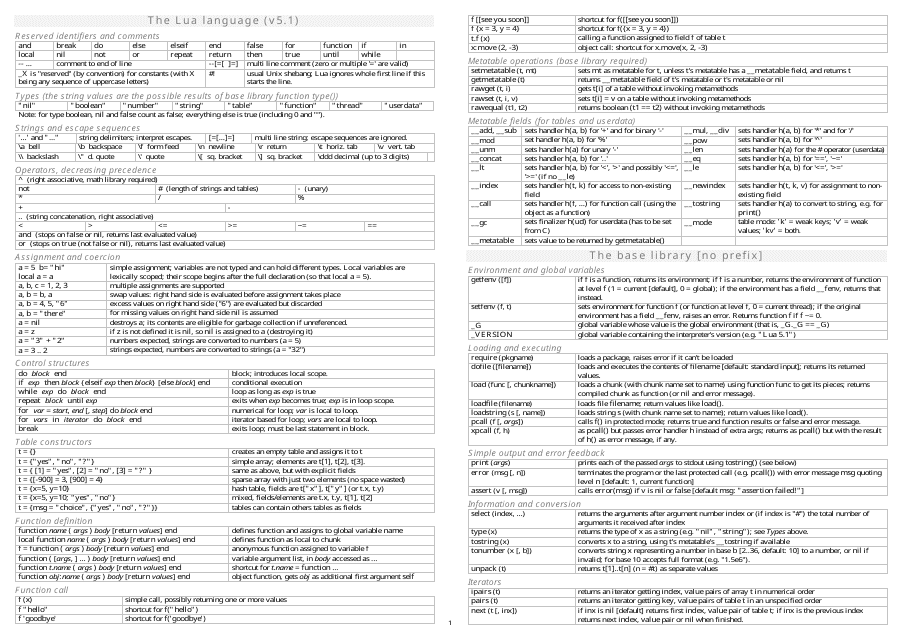
- Lua Tutorial
- Lua Basics Tutorial
- Lua Advanced
- Lua Libraries
- Lua Useful Resources
- Selected Reading
Let us start creating our first Lua program!
First Lua Program
Interactive Mode Programming
Lua provides a mode called interactive mode. In this mode, you can type in instructions one after the other and get instant results. This can be invoked in the shell by using the lua -i or just the lua command. Once you type in this, press Enter and the interactive mode will be started as shown below.
You can print something using the following statement −
Once you press enter, you will get the following output −
Default Mode Programming
Invoking the interpreter with a Lua file name parameter begins execution of the file and continues until the script is finished. When the script is finished, the interpreter is no longer active.
Let us write a simple Lua program. All Lua files will have extension .lua. So put the following source code in a test.lua file.
Assuming, lua environment is setup correctly, let’s run the program using the following code −
We will get the following output −
Let's try another way to execute a Lua program. Below is the modified test.lua file −
Here, we have assumed that you have Lua interpreter available in your /usr/local/bin directory. The first line is ignored by the interpreter, if it starts with # sign. Now, try to run this program as follows −
We will get the following output.
Let us now see the basic structure of Lua program, so that it will be easy for you to understand the basic building blocks of the Lua programming language.
Tokens in Lua
A Lua program consists of various tokens and a token is either a keyword, an identifier, a constant, a string literal, or a symbol. For example, the following Lua statement consists of three tokens −
The individual tokens are −
Comments
Comments are like helping text in your Lua program and they are ignored by the interpreter. They start with --[[ and terminates with the characters --]] as shown below −
Lua Cheat Sheet Pdf
Identifiers
A Lua identifier is a name used to identify a variable, function, or any other user-defined item. An identifier starts with a letter ‘A to Z’ or ‘a to z’ or an underscore ‘_’ followed by zero or more letters, underscores, and digits (0 to 9).
Lua does not allow punctuation characters such as @, $, and % within identifiers. Lua is a case sensitive programming language. Thus Manpower and manpower are two different identifiers in Lua. Here are some examples of the acceptable identifiers −
Keywords
The following list shows few of the reserved words in Lua. These reserved words may not be used as constants or variables or any other identifier names.
and | break | do | else |
elseif | end | false | for |
function | if | in | local |
nil | not | or | repeat |
return | then | true | until |
while |
Whitespace in Lua
A line containing only whitespace, possibly with a comment, is known as a blank line, and a Lua interpreter totally ignores it.
Lua Tutorial
Whitespace is the term used in Lua to describe blanks, tabs, newline characters and comments. Whitespace separates one part of a statement from another and enables the interpreter to identify where one element in a statement, such as int ends, and the next element begins. Therefore, in the following statement −
There must be at least one whitespace character (usually a space) between local and age for the interpreter to be able to distinguish them. On the other hand, in the following statement −
No whitespace characters are necessary between fruit and =, or between = and apples, although you are free to include some if you wish for readability purpose.
